Stop Guessing, Start Debugging: Your Quick Guide to VS Code for Node.js
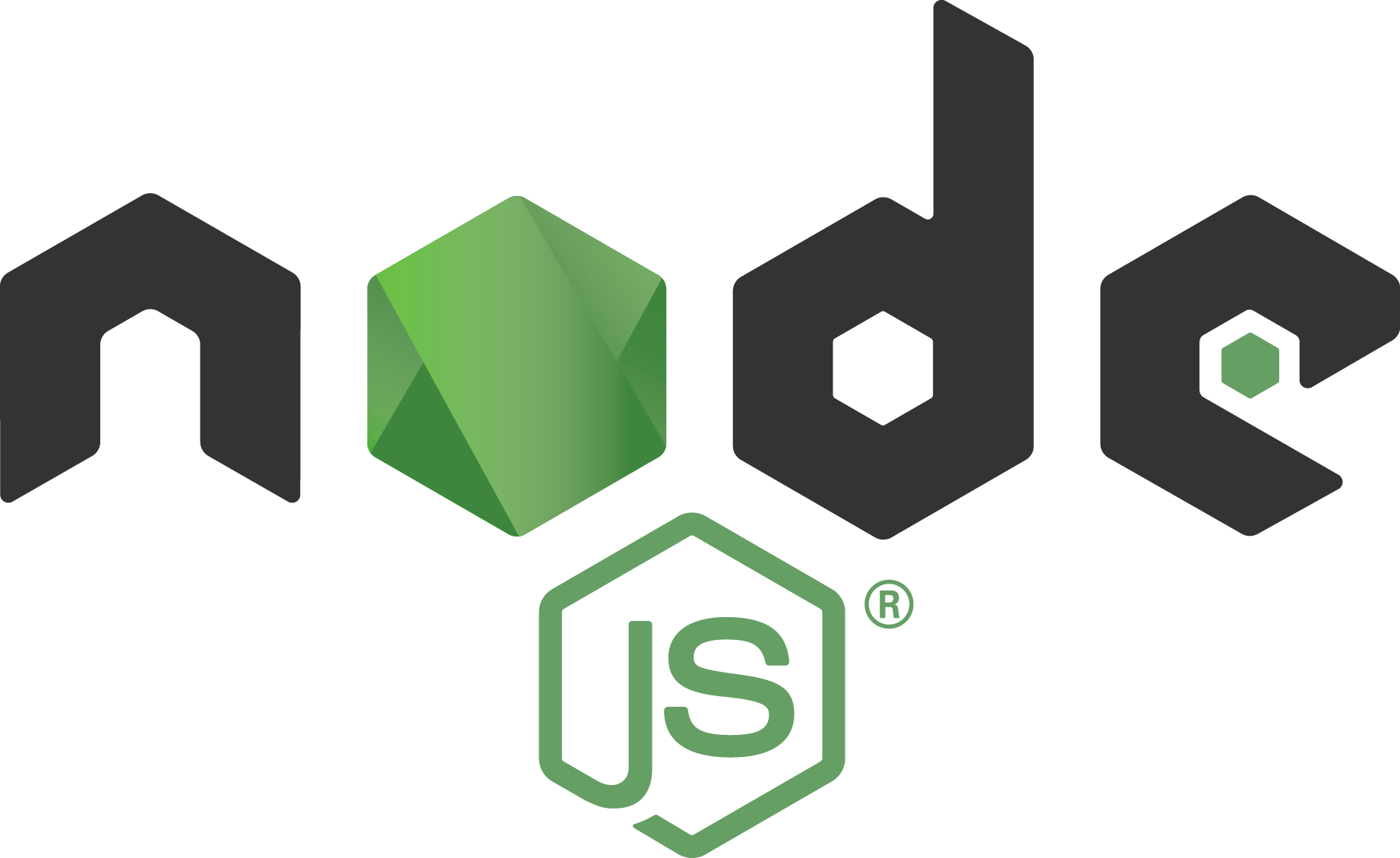
Introduction
Tired of endless console.log() statements when your Node.js app misbehaves? VS Code offers powerful debugging tools right at your fingertips, making it easier and faster to find and fix those pesky bugs. This short guide will get you started with the essentials.
Quick Setup: Your launch.json
VS Code uses a launch.json file to configure debugging. If you don't have one, go to the Debug view (the bug icon on the left) and click "create a launch.json file." Choose "Node.js." This basic configuration will allow you to debug your main application file.
{
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Launch Program",
"program": "${workspaceFolder}/app.js" // Replace with
your main file
}
]
}
Breakpoints: Pause and Inspect
The most fundamental debugging tool is the breakpoint. Simply click in the gutter (the space to the left of the line numbers) next to the line of code where you want to pause execution. A red dot will appear. Run your app in debug mode (F5). When your code reaches that line, it will stop.
Essential Stepping Controls 🙋
- Continue: Move to the next breakpoint or the end of the program.
- Step Over: Execute the current line and move to the next line without going into functions.
- Step Into: Go inside a function call on the current line.
- Step Out: Finish the current function and return to where it was called.
Use these controls in the Debug toolbar (appears when debugging) to navigate your code step by step.
Inspecting Variables
While paused at a breakpoint, the "Variables" pane in the Debug sidebar shows you the current values of variables in the current scope. Expand objects and arrays to see their contents. This is invaluable for understanding the state of your application.
The Debug Console
The "Debug Console" (View -> Debug Console) allows you to:
-
See output from
console.log()
statements. - Evaluate expressions on the fly by typing them in and pressing Enter.
Quick Tips for Effective Debugging
- Be strategic with breakpoints: Focus on the area where you suspect the issue.
- Use "Watch" for specific variables: Add expressions to the "Watch" pane to track their values across steps.
- Don't be afraid to step into functions: Sometimes the problem lies within a called function.
Summary
Debugging doesn't have to be a frustrating guessing game. VS Code provides powerful tools to help you understand your Node.js code's execution flow and identify bugs quickly. Take a few minutes to familiarize yourself with breakpoints and stepping controls, and you'll significantly improve your development workflow. Happy debugging!